티스토리 뷰
[OCPJP]Java SE 8 Programmer II Certification Exam | 1Z0-809 덤프/DUMP 문제#41~60
혲이. 2021. 3. 1. 22:04NEW QUESTION 41
In 2015, daylight saving time in New York, USA, begins on March 8th at 2:00 AM. As a result, 2:00 AM becomes 3:00 AM. Given the code fragment:
1 2 3 4 5 |
ZoneId zone = ZoneId.of("America/New_York"); ZonedDateTime dt = ZonedDateTime.of(LocalDate.of(2015, 3, 8), LocalTime.of(1, 0), zone); ZonedDateTime dt2 = dt.plusHours(2); System.out.print(DateTimeFormatter.ofPattern("H:mm - ").format(dt2)); System.out.println("difference: " + ChronoUnit.HOURS.between(dt, dt2)); |
Which is the result?
A. 3:00 – difference: 2
B. 2:00 – difference: 1
C. 4:00 – difference: 3
D. 4:00 – difference: 2
Answer: B
Explanation:
LocalTime.of(1, 0) : 1시 0분을 의미함
2015년 3월 8일이 미국 썸머타임이라서 2시간을 더했지만, 4시가 된다.
NEW QUESTION 42
Given the code fragment:
1 2 3 4 |
ZonedDateTime depart = ZonedDateTime.of(2015, 1, 15, 3, 0, 0, 0, ZoneId.of("UTC-7")); ZonedDateTime arrive = ZonedDateTime.of(2015, 1, 15, 9, 0, 0, 0, ZoneId.of("UTC-5")); long hrs = ChronoUnit.HOURS.between(depart, arrive); //line n1 System.out.println("Travel time is" + hrs + "hours"); |
What is the result?
A. Travel time is 4 hours
B. Travel time is 6 hours
C. Travel time is 8 hours
D. An exception is thrown at line n1.
Answer: A
Explanation: 한국시간이 UTC+9 이다.
UTC는 한국시간에서 -9와 같다
UTC-7은 한국시간에서 -9-7과 같다.
UTC-5는 한국시간에서 -9-5와 같다.
depart가 3시(UTC기준:3+7= 10)
arrive가 9시(UTC기준: 9+5 =14)
이므로 차이는 4시간이다.
NEW QUESTION 43
Given that data.txt and alldata.txt are accessible, and the code fragment:
1 2 3 4 5 6 7 8 9 |
public void writeFiles() throws IOException{ BufferedReader br = new BufferedReader(new FileReader("data.txt")); BufferedWriter bw = new BufferedWriter(new FileWriter("alldata.txt")); String line = null; while((line = br.readLine()) != null){ bw.append(line + "\n"); } //line n1 } |
What is required at line n1 to enable the code to overwrite alldata.txt with data.txt?
A. br.close();
B. bw.writeln();
C. br.flush();
D. bw.flush();
Answer: D
Explanation: BufferedWriter 의 경우 버퍼를 잡아 놓았기 때문에 반드시 flush() / close() 를 반드시 호출해 주어 뒤처리를 해주어야합니다.
NEW QUESTION 44
Given the code fragment:
1 2 3 4 |
String str = "Java is a programming language"; ToIntFunction<String> indexVal = str::indexOf; //line n1 int x = indexVal.applyAsInt("Java"); //line n2 System.out.println(x); |
What is the result?
A. 0
B. 1
C. A compilation error occurs at line n1.
D. A compilation error occurs at line n2.
Answer: A
NEW QUESTION 45
Given:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
interface Interface1 { public default void sayHi(){ System.out.println("Hi Interface-1"); } } interface Interface2{ public default void sayHi(){ System.out.println("Hi Interface-2"); } } public class MyClass implements Interface1, Interface2{ public static void main(String[] args){ Interface1 obj = new MyClass(); obj.sayHi(); } public void sayHi(){ System.out.println("Hi MyClass"); } } |
What is the result?
A. Hi Interface-2
B. A compilation error occurs.
C. Hi Interface-1
D. Hi MyClass
Answer: D
NEW QUESTION 46
Given:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
class Student{ String course, name, city; public Student(String name, String course, String city){ this.course = course; this.name = name; this.city = city; } public String toString(){ return course + ":" + name + ":" + city; } public String getCourse(){return course;} public String getName(){return name;} public String getCity(){return city;} } |
and the code fragment:
1 2 3 4 5 6 7 8 9 |
List<Student> stds = Arrays.asList( new Student("Jessy", "Java ME", "Chicago"), new Student("Helen", "Java EE", "Houston"), new Student("Mark", "Java ME", "Chicago") ); stds.stream() .collect(Collectors.groupingBy(Student::getCourse)) .forEach((src, res) -> System.out.println(src));
|
What is the result?
A. [Java EE: Helen:Houston]
[Java ME: Jessy:Chicago, Java ME: Mark:Chicago]
B. Java EE
Java ME
C. [Java ME: Jessy:Chicago, Java ME: Mark:Chicago]
[Java EE: Helen:Houston]
D. A compilation error occurs.
Answer: B
Explanation: forEach((src, res) -> System.out.println(src));에서 src를 출력했으므로 B이다. res를 출력했다면, A가 출력된다.
NEW QUESTION 47
Given:
1 2 3 |
public interface LengthValidator{ public boolean checkLength(String str); } |
and
1 2 3 4 5 6 7 8 9 |
public class Txt{ public static void main(String[] args){ boolean res = new LengthValidator(){ public boolean checkLength(String str){ return str.length() > 5 && str.length() < 10; } }.checkLength("Hello"); } } |
Which interface from the java.util.function package should you use to refactor the class Txt?
A. Consumer
B. Predicate
C. Supplier
D. Function
Answer: B
Explanation:
refactor(리펙토링)이란 기존의 코드가 지니고 있는 역할은 그대로 두고 코드를 수정, 재구성 하는것이다.
예시)
1 2 3 4 5 6 7 8 |
public static Predicate hasLengthOf = new Predicate() { @Override public boolean test(String str) { return str.length() > 5 && str.length() < 10; } };
|
NEW QUESTION 48
Given:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
public class Canvas implements Drawable { public void draw() {} } public abstract class Board extends Canvas {} public class Paper extends Canvas { protected void draw(int color) {} } public class Frame extends Canvas implements Drawable { public void resize() {} } public interface Drawable { public abstract void draw(); } |
Which statement is true?
A. Board does not compile.
B. Paper does not compile.
C. Frame does not compile.
D. Drawable does not compile.
E. All classes compile successfully.
Answer: E
Explanation: 인터페이스의 모든 메소드는 기본적으로 public 접근 제한자를 갖기 때문에 구현 클래스에서 작성할 때 public 보다 더 강한 접근 제한자(default, private 등)를 사용할 수 없다. 즉, public을 생략해선 안된다. interface 를 구현해서 메소드를 오버라이딩 할 때는 반드시 접근 제한자를 public 으로 해야 한다.
오버라이딩 시, 부모 클래스의 메소드의 접근 제한자보다 좁아질 수 없다. 확장될 수는 있다.
오버로딩 시, 반환 자료형, 접근 제한자는 달라도 된다. (오버로딩에 영향을 미치지 않는다.)
NEW QUESTION 49
Given the code fragment:
1 2 3 4 |
Stream < List < String >> iStr = Stream.of( Arrays.asList("1", "John"), Arrays.asList("2", null) ); Stream < String > nInSt = iStr.flatMapToInt((x) -> x.stream()); nInSt.forEach(System.out::print); |
What is the result?
A. 1John2null
B. 12
C. A NullPointerException is thrown at run time.
D. A compilation error occurs.
Answer: D
Explanation: iStr은 Stream<String>이므로 flatMapToInt에서 컴파일 에러가 난다.
Stream<String> cannot be converted to IntStream
NEW QUESTION 50
Given the code fragment:
1 2 3 4 5 6 |
List < String > colors = Arrays.asList("red", "green", "yellow"); Predicate < String > test = n ->{ System.out.println("Searching..."); return n.contains("red"); }; colors.stream().filter(c ->c.length() > 3).allMatch(test); |
What is the result?
A. Searching...
B. Searching...Searching...
C. Searching...Searching... Searching...
D. A compilation error occurs.
Answer: A
Explanation: allMatch() : 해당 스트림의 모든 요소가 특정 조건을 만족할 경우에 true를 반환함.
NEW QUESTION 51
Given the code fragment:
1 2 3 4 |
List < Integer > codes = Arrays.asList(10, 20); UnaryOperator < Double > uo = s ->s + 10.0; codes.replaceAll(uo); codes.forEach(c ->System.out.println(c)); |
What is the result?
A. 20.030.0
B. 1020
C. A compilation error occurs.
D. A NumberFormatException is thrown at run time.
Answer: C
Explanation: UnaryOperator<Double> cannot be converted to UnaryOperator<Integer>
NEW QUESTION 52
Given:
1 2 3 4 5 6 7 8 9 |
interface Rideable { Car getCar(String name); } class Car { private String name; public Car(String name) { this.name = name; } } |
Which code fragment creates an instance of Car?
A. Car auto = Car ("MyCar")::new;
B. Car auto = Car::new;
Car vehicle = auto::getCar("MyCar");
C. Rideable rider = Car::new;
Car vehicle = rider.getCar("MyCar");
D. Car vehicle = Rideable::new::getCar("MyCar");
Answer: C
Explanation: ..
NEW QUESTION 53
Given the code fragment:
1 2 3 |
List < Integer > nums = Arrays.asList(10, 20, 8); System.out.println( //line n1 ); |
Which code fragment must be inserted at line n1 to enable the code to print the maximum number in the nums list?
A. nums.stream().max(Comparator.comparing(a -> a)).get()
B. nums.stream().max(Integer : : max).get()
C. nums.stream().max()
D. nums.stream().map(a -> a).max()
Answer: A
NEW QUESTION 54
Given the definition of the Country class:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
public class country { public enum Continent { ASIA, EUROPE } String name; Continent region; public Country(String na, Continent reg) { name = na, region = reg; } public String getName() { return name; } public Continent getRegion() { return region; } } |
and the code fragment:
1 2 3 4 5 6 7 8 9 |
List < Country > couList = Arrays.asList( new Country("Japan", Country.Continent.ASIA), new Country("Italy", Country.Continent.EUROPE), new Country("Germany", Country.Continent.EUROPE) ); Map < Country.Continent, List < String >> regionNames = couList.stream() .collect(Collectors.groupingBy(Country::getRegion, Collectors.mapping(Country::getName, Collectors.toList()))); System.out.println(regionNames); |
A. {EUROPE = [Italy, Germany], ASIA = [Japan]}
B. {ASIA = [Japan], EUROPE = [Italy, Germany]}
C. {EUROPE = [Germany, Italy], ASIA = [Japan]}
D. {EUROPE = [Germany], EUROPE = [Italy], ASIA = [Japan]}
Answer: B
NEW QUESTION 55
Given the code fragment:
1 2 3 |
//line n1 Double d = str.average().getAsDouble(); System.out.println("Average = " + d); |
Which should be inserted into line n1 to print Average = 2.5?
A. IntStream str = Stream.of (1, 2, 3, 4);
B. IntStream str = IntStream.of (1, 2, 3, 4);
C. DoubleStream str = Stream.of (1.0, 2.0, 3.0, 4.0);
D. Stream str = Stream.of (1, 2, 3, 4);
Answer: C
NEW QUESTION 56
Given:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
class RateOfInterest { public static void main(String[] args) { int rateOfInterest = 0; String accountType = "LOAN"; switch (accountType) { case "RD"; rateOfInterest = 5; break; case "FD"; rateOfInterest = 10; break; default: assert false: "No interest for this account"; //line n1 } System.out.println("Rate of interest:" + rateOfInterest); } } |
and the command:
java –ea RateOfInterest
What is the result?
A. Rate of interest: 0
B. An AssertionError is thrown.
C. No interest for this account
D. A compilation error occurs at line n1.
Answer: B
Explanation:
Java에서 단언문 assert는 JDK 1.4 부터 지원합니다. 객체가 아니고 예약어 입니다.
사용법은 두 가지 형식이 있는데, 다음과 같습니다.
assert expression1;
assert expression1: expression2;
첫 번째는 인자로 boolean으로 평가되는 표현식 또는 값을 받아서 참이면 그냥 지나가고, 거짓이면 AssertionError 예외가 발생합니다. 두 번째는 표현식1이 거짓인 경우 두번째 표현식의 값이 예외 메세지로 보여지게 됩니다.
Assertion은 디버깅 용도로 사용되어서 JVM 기본 설정으로 프로그램을 실행하게 되면 assert문은 모두 실행에서 제외 됩니다. assert가 동작하게 하려면 실행시 -ea 옵션을 사용해서 실행합니다.
java -ea 클래스명
NEW QUESTION 57
Given the code fragment:
1 2 3 4 5 |
public static void main(String[] args){ Console console = System.console(); char[] pass = console.readPassword("Enter password:");//line n1 String password = new String(pass);//line n2 } |
What is the result?
A. A compilation error occurs at line n1.
B. A compilation error occurs at line n2.
C. The code reads the password without echoing characters on the console.
D. A compilation error occurs because the IOException isn’t declared to be thrown or caught?
Answer: A
Explanation:
char[] |
readPassword() |
Reads a password or passphrase from the console with echoing disabled |
char[] |
readPassword(String fmt, Object... args) |
Provides a formatted prompt, then reads a password or passphrase from the console with echoing disabled. |
NEW QUESTION 58
Given:
Item table
• ID, INTEGER: PK
• DESCRIP, VARCHAR(100)
• PRICE, REAL
• QUANTITY< INTEGER
And given the code fragment:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
try { Connection conn = DriveManager.getConnection(dbURL, username, password); String query = "Select * FROM Item WHERE ID = 110"; Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery(query); while (rs.next()) { System.out.println("ID: " + rs.getInt("Id")); System.out.println("Description: " + rs.getString("Descrip")); System.out.println("Price: " + rs.getDouble("Price")); System.out.println("Quantity: " + rs.getInt("Quantity")); } } catch(SQLException se) { System.out.println("Error"); } |
Assume that:
The required database driver is configured in the classpath.
The appropriate database is accessible with the dbURL, userName, and passWord exists.
The SQL query is valid. What is the result?
A. An exception is thrown at runtime.
B. Compilation fails.
C. The code prints Error.
D. The code prints information about Item 110.
Answer: D
NEW QUESTION 59
Given the code fragment:
1 2 3 4 5 6 7 |
Deque<String> queue = new ArrayDeque<>(); queue.add("Susan"); queue.add("Allen"); queue.add("David"); System.out.println(queue.pop()); System.out.println(queue.remove()); System.out.println(queue); |
What is the result?
A. David
David
[Susan, Allen]
B. Susan
Susan
[Susan, Allen]
C. Susan
Allen
[David]
D. David
Allen
[Susan]
E. Susan
Allen
[Susan, David]
Answer: C
Explanation:
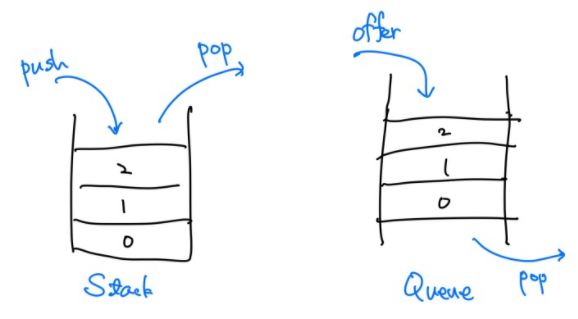
NEW QUESTION 60
You have been asked to create a ResourceBundle which uses a properties file to localize an application. Which code example specifies valid keys of menu1 and menu2 with values of File Menu and View Menu?
A. <key name = ‘menu1">File Menu</key>
<key name = ‘menu2">View Menu</key>
B. <key>menu1</key>
<value>File Menu</value>
<key>menu2</key>
<value>View Menu</value>
C. menu1, File Menu, menu2, View Menu Menu
D. menu1 = File Menu
menu2 = View Menu
Answer: D
Explanation:
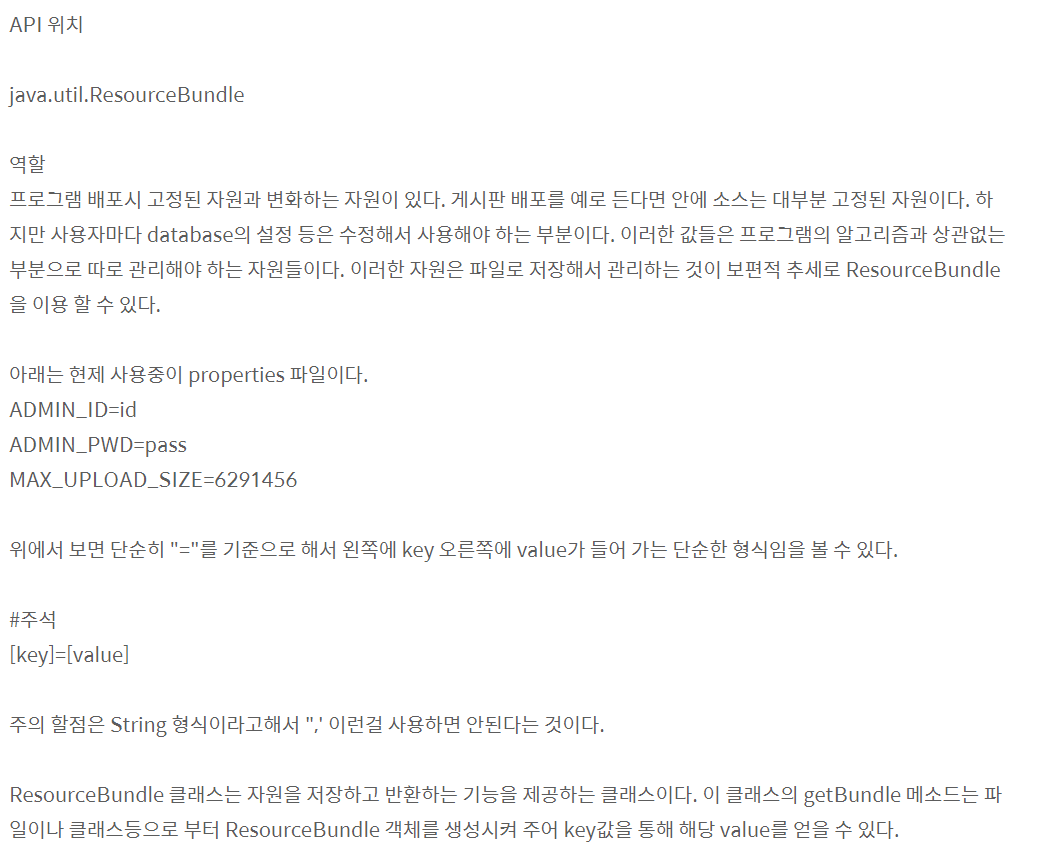
'Language > java' 카테고리의 다른 글
- Total
- Today
- Yesterday
- 백준
- 백준퇴사
- C# java 차이점
- c#
- 선언적트랜잭션 #noRollbackFor #@Transactional
- boj
- 프론트엔드
- html
- 개발중캐시삭제
- 프론트엔드개발자
- 백준14501
- html꿀팁
- script버전
- 퇴사
- 캐시삭제
- C++
- 런타임에러
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |